Multiple Inheritance
A true Object-Oriented Solar_System
The purpose of this exercise is to rewrite the previous exercise using OOP.
Remember that privacy is still important!
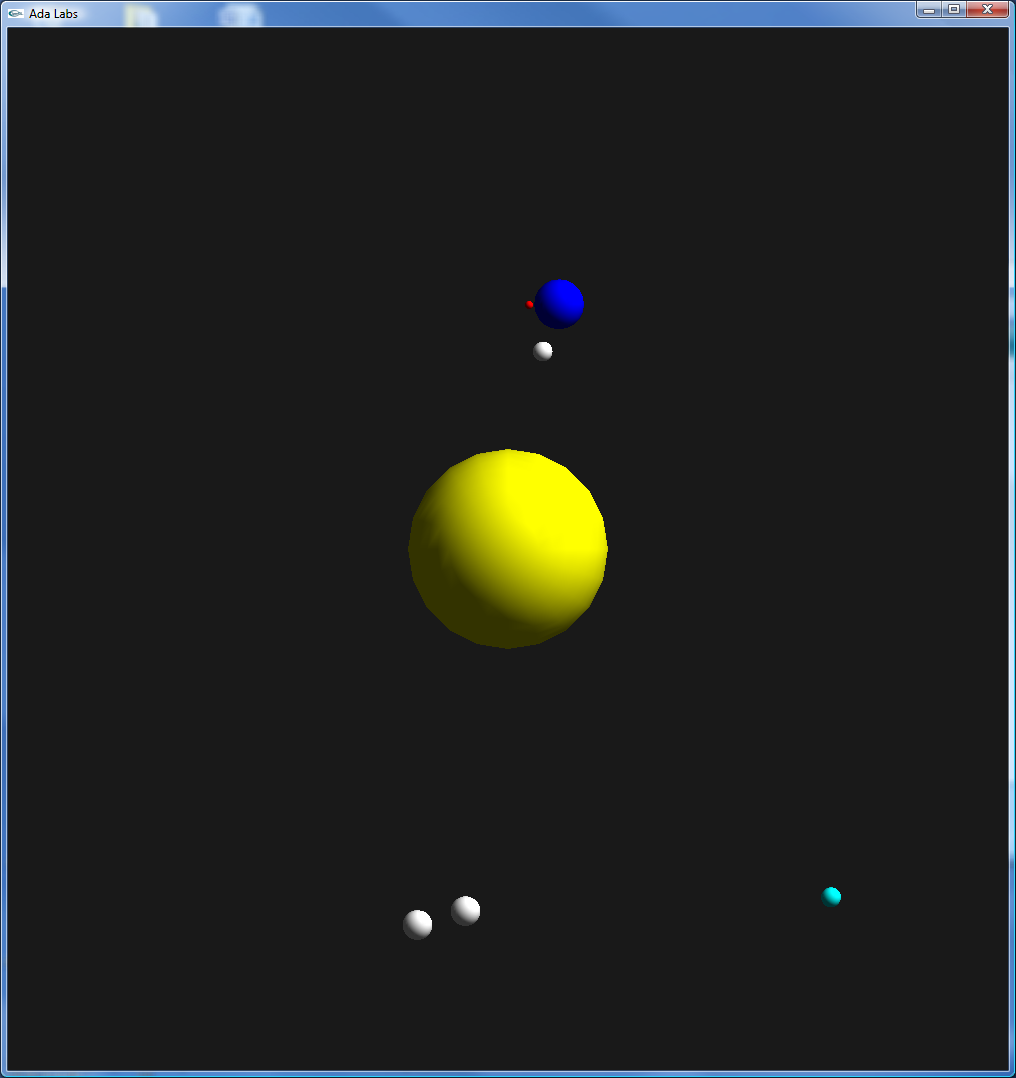
Expected result
Question 1
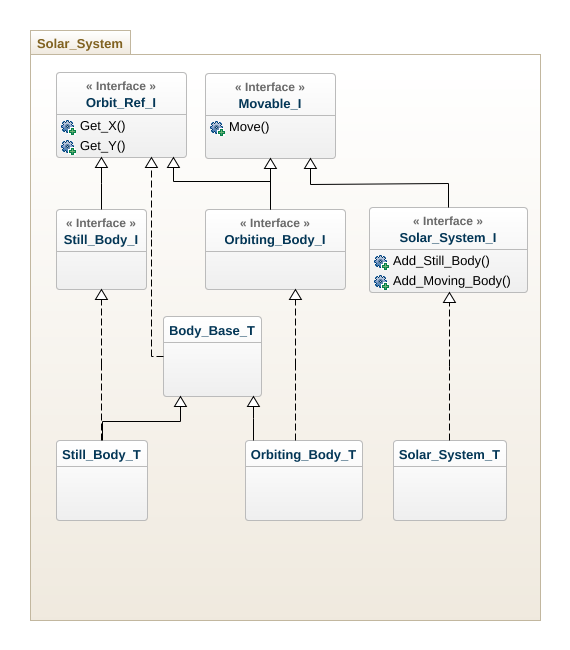
Class diagram for Q.1 and Q.2
Create a hierarchy of interfaces as follows
Orbit_Ref_I
as an interface implementingGet_X
andGet_Y
(can be used as an orbit reference)Movable_I
as an interface implementing aMove
procedureOrbiting_Body_I
as an interface implementingOrbit_Ref_I
andMovable_I
Still_Body_I
as an interface implementingOrbit_Ref_I
Solar_System_I
as an interface implementingAdd_Still_Body
andAdd_Moving_Body
procedures andMovable_I
Question 2
Create a hierarchy of tagged types as follows
Body_Base_T
to store a position (X
,Y
) and implementingOrbit_Ref_I
Orbiting_Body_T
as a concrete object extendingBody_Base_T
to storeDistance
,Speed
,Angle
andTurns_Around
and implementingOrbiting_Body_I
Still_Body_T
as a concrete object extendingBody_Base_T
and implementingStill_Body_I
Solar_System_T
as a concrete object implementingSolar_System_I
able to store a vector of moving bodies and a vector of still bodies
Question 3
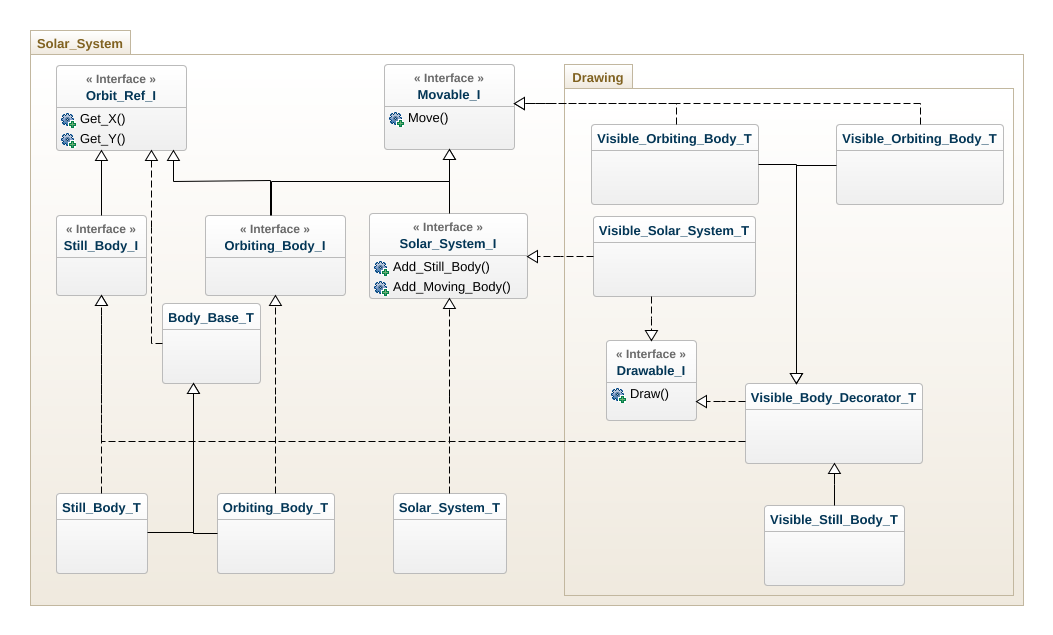
Class diagram for Q.3, Q.4 and Q.5
Add constructor primitives in order to create concrete types returning a pointer to the newly allocated object
Create_Orbiting
returning an access toOrbiting_Body_T
Create_Still
returning an access toStill_Body_T
Create_Solar_System
returning an access toSolar_System_T
Question 4
In a specific Graphics package extend the capabilities of our object using decorator design pattern.
Create an interface
Drawable_I
implementing aDraw
procedureDefine
Visible_Body_Decorator_T
as an abstract type implementingDrawable_I
andStill_Body_I
. This type will store graphic information.Define
Visible_Orbiting_Body_T
extendingVisible_Body_Decorator_T
and implementingMovable_I
Define
Visible_Still_Body_T
extendingVisible_Body_Decorator_T
Define
Visible_Solar_System_T
implementingDrawable_I
andSolar_System_I
Question 5
Add constructor
Create_Visible
toVisible_Orbiting_Body_T
Add constructor
Create_Visible
toVisible_Still_Body_T
Add constructor
Create_Visible
toVisible_Solar_System_T
Question 6
Make it work ! ;)